How to Design with PEAR Templates
Creating a Block in a PEAR template
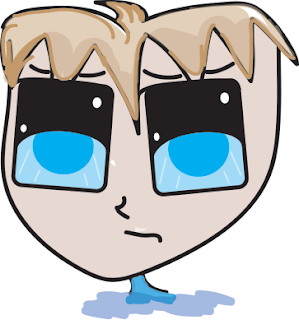
Still Not Convinced?? Why is there only one row?
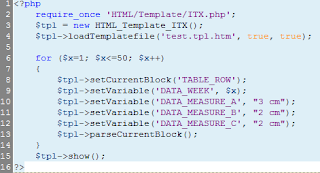
From 1 to 50 in less than 5 seconds...
Practice Makes Perfect
- Break the design into repeating "blocks."
- Anything that recurs in the design will be a new block in the PEAR template.
- Try to generalize information and set that as a variable in the PEAR template.
Creating a Block in a PEAR template
A good place to use a block might be for a list of information that we are pulling from a database. This information will then be populated into a table of statistics. Our block that we are going to create is a generic table row. We will then call this block from the PHP and loop through the data in the PHP, populating the block.
As a starting example, we will use PEAR templates, to create a template for this data table to track Plant Growth.

First, in the above table, we need to identify which information is static (not changing) and which information is dynamic. This will determine how we are going to organize the blocks and variables in the PEAR Template.
What is the Static Information?As a starting example, we will use PEAR templates, to create a template for this data table to track Plant Growth.

First, in the above table, we need to identify which information is static (not changing) and which information is dynamic. This will determine how we are going to organize the blocks and variables in the PEAR Template.
Assuming we are looking at just the table and not the title of the table, the static information is the table header information.
Simply a table row with the following header information
What is the Dynamic Information?Simply a table row with the following header information
- Week
- A (Fertilizer "X")
- B (Fertilizer "Y")
- C (No Fertilizer)
The dynamic information are the values recorded for each cell. This kind of information can most likely be stored in a database and looped through in the PHP to retrieve it from the database.
A table row with the measured values for each table cell.
How do I put it into a PEAR Template?A table row with the measured values for each table cell.
- Experiment Week
- Value of A during that week
- Value of B during that week
- Value of C during that week
- In PEAR, a Block looks like an HTML comment. You must put to start a block. and to end the block. All HTML tags between the start and end tag belong to that block and when the block is parsed in the PHP, all the HTML between those tags is displayed. If you know you want certain elements within those blocks, but don't want them to be too specific, use variables. A PEAR variable looks like {VARIABLE} and is assigned in the PHP.
- Take the Static information that you identified and code the HTML into your test.tpl.htm page.
Since you are creating a row of a table, you will just create a table, insert a table row, and put table headers for each static header in the table.
*Note - if you try to view this in the web page at this point nothing will show up because we have not added a block to the template yet.
- Create a Block for the Dynamic information in the test.tpl.htm page.
The block should consist of a row with variables to input values for the week, fertilizer a, fertilizer b, fertilizer c.
*Note - still nothing will show up after this step because we need to add the PHP to actually insert the variables and parse the block.
- Add the PHP on test.php to set the Block, set the Variables and Parse the block.
In the template, we created a block called TABLE_ROW in the test.tpl.htm file. We are going to set this block on the PHP page, and set each Variable.
Quick PEAR reference:
(Assuming your template is set to the variable $tpl which we used in this tutorial).- Set a block: $tpl->setCurrentBlock('BLOCK NAME')
- Set a Variable: $tpl->setVariable('VARIABLE NAME', "VARIABLE VALUE (CAN INCLUDE HTML)")
- Parse a block: $tpl->parseCurrentBlock()
- *Don't forget to show the template! $tpl->show()
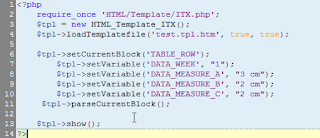

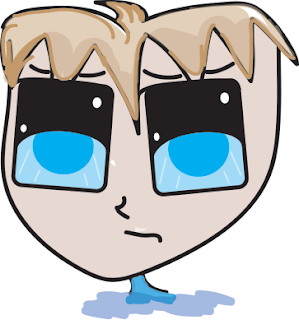
Still Not Convinced?? Why is there only one row?
Ideally this information would be pulled from a database. Unless you have recently conducted a study on plant growth in various fertilizers, I'm guessing you don't have this information readily stored on your local database. Not to say it wouldn't be a simple task to pop in some fake data. However, just to show you the concept of reusing a block in PEAR templates, I am going to run a loop in PHP, which will simulate a pull from the database. The loop will call the block and populate fake data for our fertilizer table and in a matter of seconds we will go from one to 50 records keeping the HTML completely abstracted from the logic of the website.
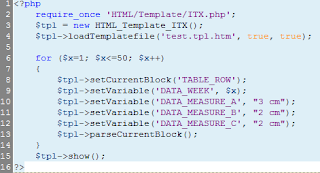
From 1 to 50 in less than 5 seconds...
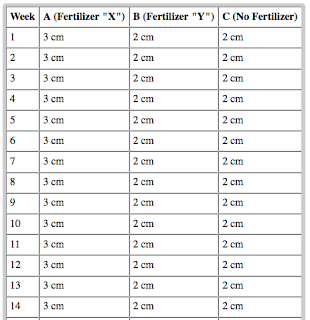
Practice Makes Perfect
This was just a very basic example of how to use PEAR Templates, not just how to program with them, but the mindset when developing with them. For the next post on this topic, I will recreate a foursquare template layout using this technique. So, a good example to practice the concept is with the following example. Try to re-create a simple periodic table using PHP and HTML PEAR templates.

Comments
Post a Comment